Creating JavaScript Templates
JavaScript Templates
JavaScript Templates enable users to define custom JavaScript functions that transform data structures while maintaining code simplicity and readability. This system provides a more powerful alternative to traditional templating solutions such as Handlebars.js and Jsonnet.
Implementation Overview
JavaScript Templates are implemented through a transformData
function that processes input data and returns a transformed JSON-able output structure. The function can be created either manually or through the AI-powered Template Co-Pilot.
Creating JavaScript Templates
UI Implementation
- Access the Template Builder
- Define the desired JSON output structure in the
Template Co-Pilot
form (Box 2) - Provide additional context for the AI Co-Pilot
- Generate the template using the
Generate Template
button - Customize the generated template in the Customize Template form
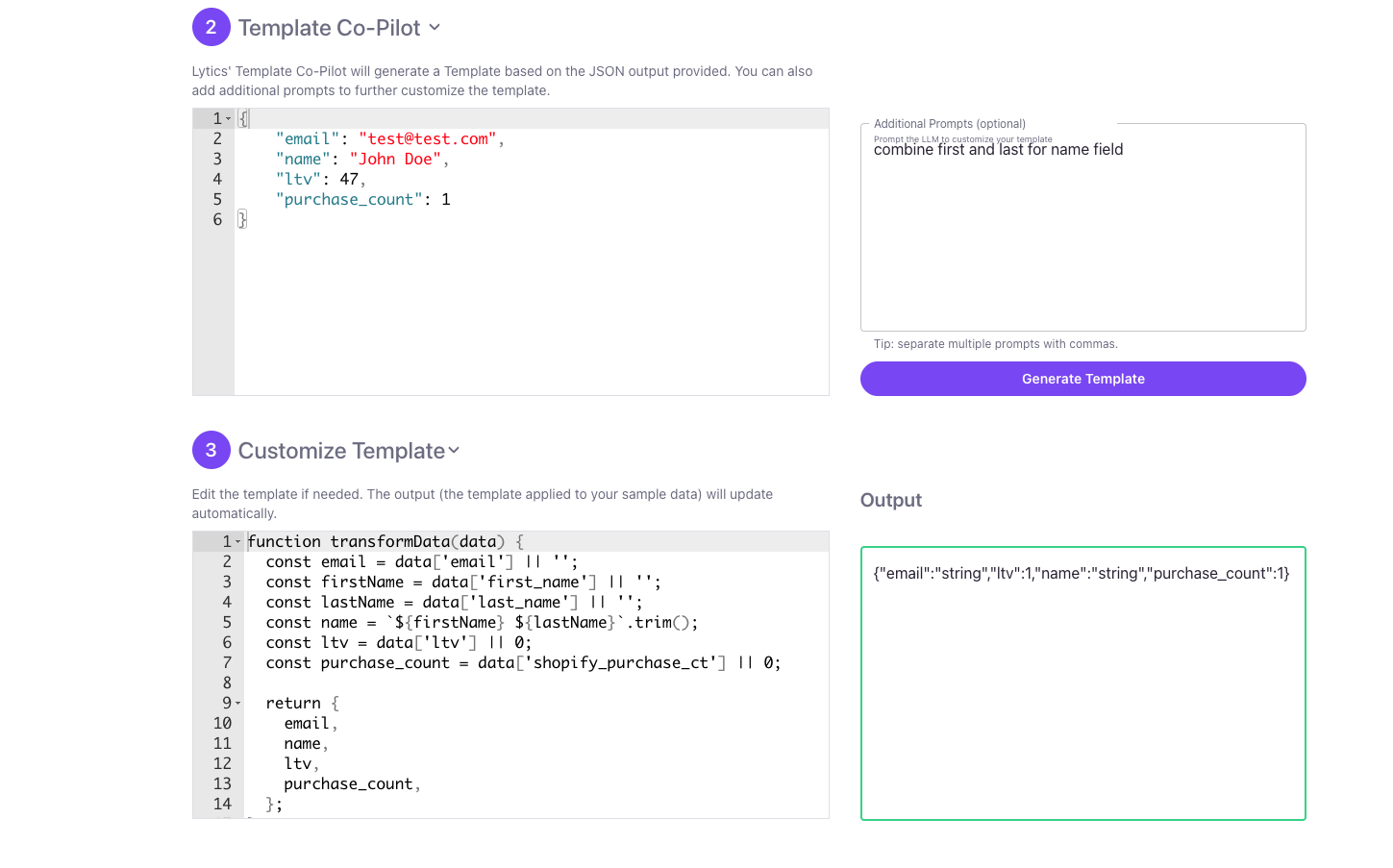
In the example above, our desired JSON output contains a users' email
, name
, ltv
, and purchase count
. To guide the AI Co-Pilot, we'll add an additional prompt to combine the first
and last
fields on the user profile to derive the name
field in the output. Once the JavaScript function is generated, the function can be edited to customize the Template.
API Implementation
Templates can be created programmatically using the Lytics API. The following example demonstrates template creation via API:
echo "function transformData(data) {
const email = data['email'] || '';
const firstName = data['first_name'] || '';
const lastName = data['last_name'] || '';
const name = `${firstName} ${lastName}`.trim();
const ltv = data['ltv'] || 0;
const purchase_count = data['shopify_purchase_ct'] || 0;
return {
email,
name,
ltv,
purchase_count,
};
}" | http https://api.lytics.io/v2/template key==<your API key> name=="JS Template" type=="js1"
Function Structure
The transformData
function follows this pattern:
function transformData(data) {
// Field extraction with fallback values
const email = data['email'] || '';
const firstName = data['first_name'] || '';
const lastName = data['last_name'] || '';
// Data transformation
const name = `${firstName} ${lastName}`.trim();
// Return transformed structure
return {
email,
name,
// Additional fields
};
}
Implementation Guidelines
Data Access
- Utilize bracket notation or dot notation for field access:
data['field_name']
ordata.field_name
- Implement fallback values for all fields
- Handle nested object access appropriately
Error Handling
- Implement graceful field absence handling
- Utilize appropriate default values
- Validate data types when necessary
Best Practices
- Maintain transformation simplicity
- Avoid complex nested operations
- Utilize efficient data structures
Troubleshooting
Common Issues
-
Missing Fields
- Implement fallback values
- Validate field existence
- Handle null/undefined cases
-
Type Errors
- Validate data types
- Implement type conversion
- Utilize appropriate fallbacks
For additional information or specific use cases, please refer to the API documentation or contact technical support.
Updated about 18 hours ago